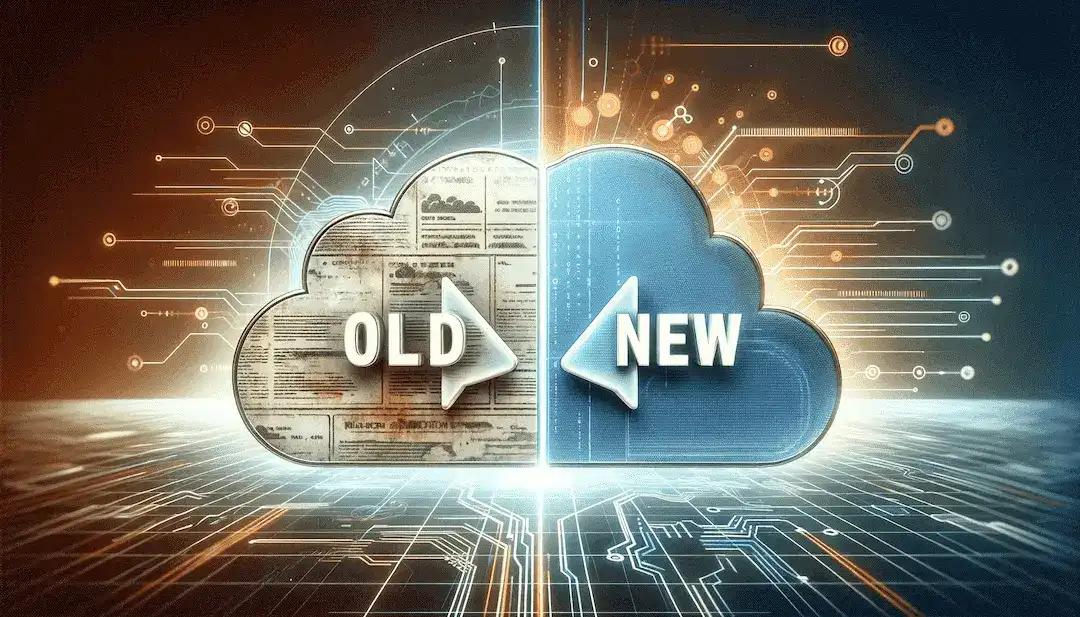
Behind the Screen: Refreshing Your Finance Wisdom on a Cached Web
Today, I want to share a little behind-the-scenes story from my own blogger’s toolbox – something I consider might help you in your digital ventures.
Hey there, tech enthusiasts and curious minds!
Today, we're diving into a common but tricky issue many of us face while dealing with web development: the dreaded CORS error. Don’t worry; I’ll keep the tech babble to a minimum and explain things in a way everyone can understand.
So, let's set the scene. You've written some AJAX code to send data to AWS API Gateway. But, instead of smooth sailing, you're greeted with an error message about CORS policy blocking your request. Sounds frustrating, right? Let's decode this in simple terms.
AJAX Code:
$.ajax({
type: "POST",
url : "https://xxxx.execute-api.region.amazonaws.com/",
dataType: "json",
contentType: "application/json; charset=utf-8",
data: JSON.stringify(data),
success: function () {
// Success logic
},
error: function () {
// Error logic
}
});
CORS, or Cross-Origin Resource Sharing, is like a security guard for web browsers. It's there to make sure that a web page from one site (origin) can safely access resources from another site without any security risks. Imagine you’re at a concert, and the security guard only lets people with valid tickets (from a known source) in. CORS works similarly.
The error message I encountered says, "Request header field access-control-allow-origin is not allowed by Access-Control-Allow-Headers in preflight response." This is a fancy way of saying that the security guard (CORS policy) isn't happy with the credentials (headers), AJAX request is showing. It's like trying to enter the concert with a backstage pass when you only have a general admission ticket.
Now, how do we solve this? The key lies in understanding what's going wrong. AJAX code is trying to tell the server, "Hey, I can access resources from anywhere!" But the server, which is more cautious, says, "Not so fast, I need to check if that's okay."
Here are some steps you can take to solve this issue:
Modify the Server-Side Configuration: Ensure that your AWS API Gateway configuration includes the necessary CORS headers. You should not set the CORS headers (Access-Control-Allow-Origin, etc.) on the client side but rather on the server side. AWS API Gateway can return these headers for you if configured correctly.
1. Go to the AWS API Gateway console.
2. Select your API.
3. Select the relevant method. (GET, POST etc..)
4. Click on "Actions" and select "Enable CORS".
Deploy the API: After making changes to the CORS configuration in API Gateway, make sure you redeploy your API to apply these changes.
Remove the CORS Headers from Client Request: You should not include CORS headers in your client-side request. These are response headers, not request headers.
I updated the API gateway to include CORS headers and removed these headers from the client-side request. Despite this, a new issue has emerged: the request is being blocked by the CORS policy because the 'Access-Control-Allow-Origin' header is missing from the requested resource, as indicated by 'null'.
Check the Lambda Function (if used): If your API Gateway method is integrating with a Lambda function, make sure that the Lambda function itself is returning the necessary CORS headers. The headers need to be included in the response object that the Lambda function returns.
{
'statusCode': 200,
'headers': {
'Access-Control-Allow-Origin': '*', # Or a specific domain
'Access-Control-Allow-Credentials': True # If needed
},
'body': json.dumps(body_content)
}
Once you make these tweaks, your AJAX request should work seamlessly with AWS API Gateway, and the CORS error will be a thing of the past. Remember, dealing with CORS is all about ensuring secure communication between different origins. It might seem like a hassle, but it's there for good reasons.
So, there you have it! I hope this clears up the confusion around CORS and helps you get back on track with your web development journey. Happy coding!
Today, I want to share a little behind-the-scenes story from my own blogger’s toolbox – something I consider might help you in your digital ventures.
Let's chat about something that's creating quite a buzz in tech circles – the AI Pin by Humane. It's not just another gadget